The ITS Customer Support Center supports the USC community with equipment, software, connectivity, and applications. Please note that some requests may require a government ID for user verification.
For support from an administrative or academic unit or department, check out the list of local IT contacts. Media inquiries should contact its-news@usc.edu.
Need Help?
Submit a Ticket
Phone Support
213-740-5555
Hours: 24 hours per day, 7 days per week
Report Security Issues or Suspicious Activity
To Report an Information Security Incident
Email security@usc.edu or call the ITS 24×7 support hotline 213-740-5555.
To Report Suspicious Emails
Email phishing@usc.edu
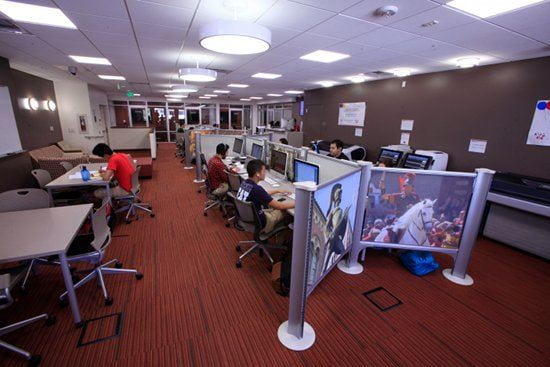
USC Computing Centers
ITS Customer Feedback
If you would like to provide feedback to the ITS Service Desk, please fill out the ITS Customer Feedback Form.
ITS Support
- The ITS Service Desk provides support to the USC community 24 hours a day, 365 days a year.
- Call (213) 740-5555 and choose option 2 to receive assistance. Please note that some requests may require a government ID for user verification.
- Report an Issue on the ITS Service Portal.
- Search for information on the ITS Service Portal.
ITS Communications
For media requests, feedback, or updates to ITS websites, please contact the ITS Communications Department.
(Note: This is not the Customer Support Center.)